如何在 C# 中将 Microsoft Word 转换为 PDF;
DOCX 文件是在 Microsoft Word 中创建的文档,Microsoft Word 是微软的文字处理程序。 它使用Office Open XML(OOXML)标准化,使其高效且与各种软件兼容。 自 Word 2007 起,它已成为 Word 文档的默认格式,替代了旧的 DOC 格式。
IronPDF允许您将DOCX文档转换为PDF,并提供邮件合并功能,用于为个别收件人生成个性化的文档批次。 将DOCX转换为PDF可确保通用兼容性,保留格式,并增加了一层安全性。
开始使用IronPDF
立即在您的项目中开始使用IronPDF,并享受免费试用。
如何在 C&num 中将 DOCX 转换为 PDF;
- 下载将 DOCX 转换为 PDF 的 C# 库
- 准备要转换的 DOCX 文件
- 实例化 DocxToPdf 渲染器 从 DOCX 文件渲染 PDF 的类
- 使用
渲染文档为 PDF
方法,并提供 DOCX 文件路径 - 利用邮件合并功能生成一批文件
将 DOCX 文件转换为 PDF 示例
要将 Microsoft Word 文件转换为 PDF,请实例化 DocxToPdfRenderer 类。 使用 DocxToPdfRenderer 对象的 RenderDocxAsPdf
方法,并提供 DOCX 文件的文件路径。此方法返回一个 PdfDocument 对象,让您可以进一步自定义 PDF。 我使用了Microsoft Word中的“现代按时间顺序排列的简历”模板作为示例。 您可以下载现代按时间顺序排列的简历 DOCX 示例文件.
Microsoft Word 预览
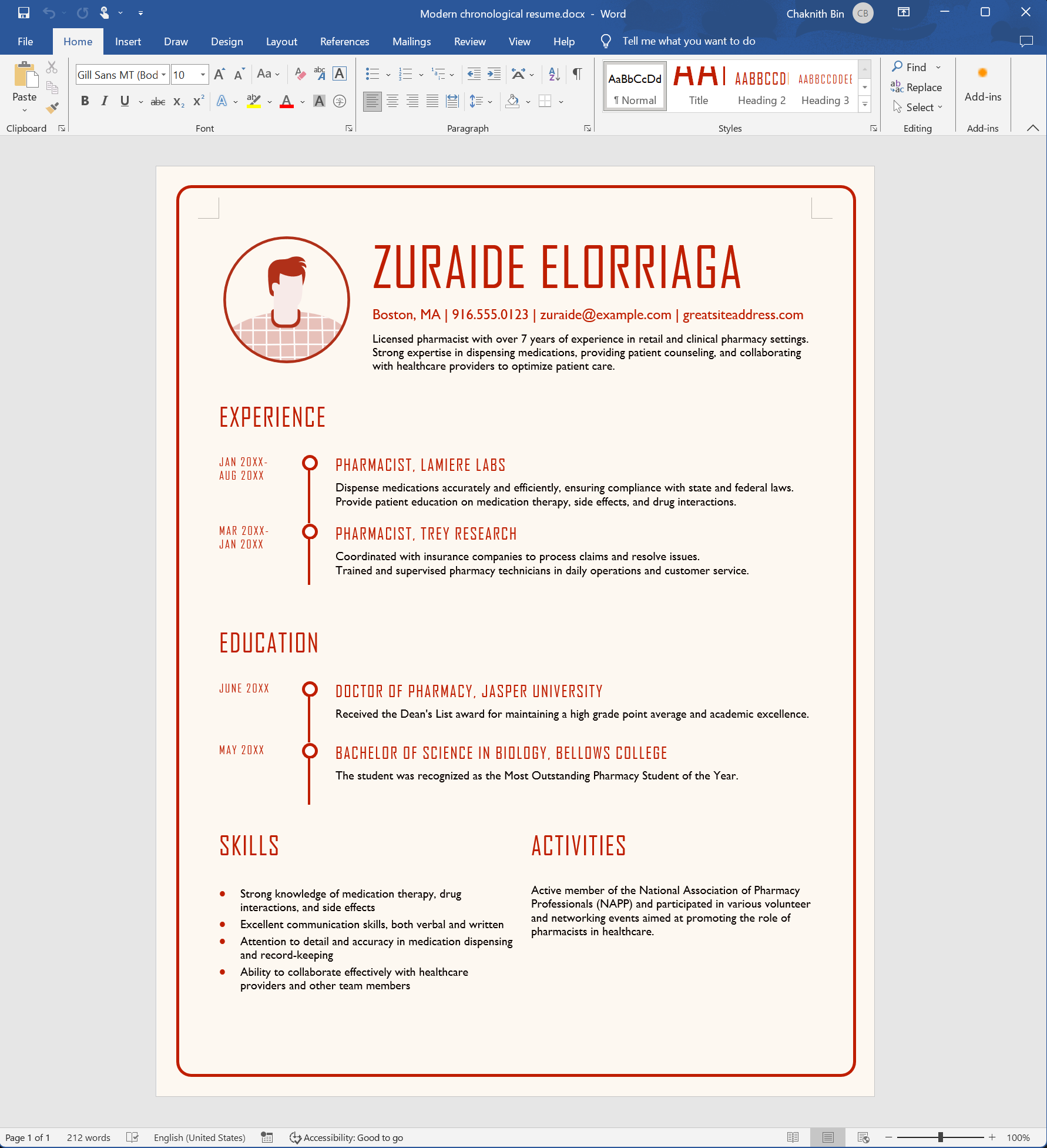
代码样本
此外,RenderDocxAsPdf
方法还接受以字节和流形式的 DOCX 数据。
:path=/static-assets/pdf/content-code-examples/how-to/docx-to-pdf-from-file.cs
using IronPdf;
// Instantiate Renderer
DocxToPdfRenderer renderer = new DocxToPdfRenderer();
// Render from DOCX file
PdfDocument pdf = renderer.RenderDocxAsPdf("Modern-chronological-resume.docx");
// Save the PDF
pdf.SaveAs("pdfFromDocx.pdf");
Imports IronPdf
' Instantiate Renderer
Private renderer As New DocxToPdfRenderer()
' Render from DOCX file
Private pdf As PdfDocument = renderer.RenderDocxAsPdf("Modern-chronological-resume.docx")
' Save the PDF
pdf.SaveAs("pdfFromDocx.pdf")
输出 PDF
邮件合并示例
邮件合并位于Microsoft Word中的“邮件”选项卡上,允许您为每个收件人或数据条目创建一批带有个性化信息的文档。 它通常用于生成个性化的信件、信封、标签或电子邮件消息,例如邀请函、新闻通讯或表格信件,其中大部分内容相同,但某些细节会因每个收件人而有所不同。
模型
首先,让我们创建一个模型来存储将要与其对应占位符进行邮件合并的信息。
:path=/static-assets/pdf/content-code-examples/how-to/docx-to-pdf-mail-merge-model.cs
internal class RecipientsDataModel
{
public string Date { get; set; }
public string Location{ get; set; }
public string Recipients_Name { get; set; }
public string Contact_Us { get; set; }
}
Friend Class RecipientsDataModel
Public Property [Date]() As String
Public Property Location() As String
Public Property Recipients_Name() As String
Public Property Contact_Us() As String
End Class
我已经修改了微软Word提供的模板以满足我们的需求。 请下载派对邀请 DOTX 示例文件. 对于我们的用例,让我们将 MailMergePrintAllInOnePdfDocument 属性设置为 true,这样可以将多个 PDF 合并为一个 PdfDocument 对象。 我们将要使用的合并字段是日期、位置、收件人姓名和联系我们。
Microsoft Word 预览
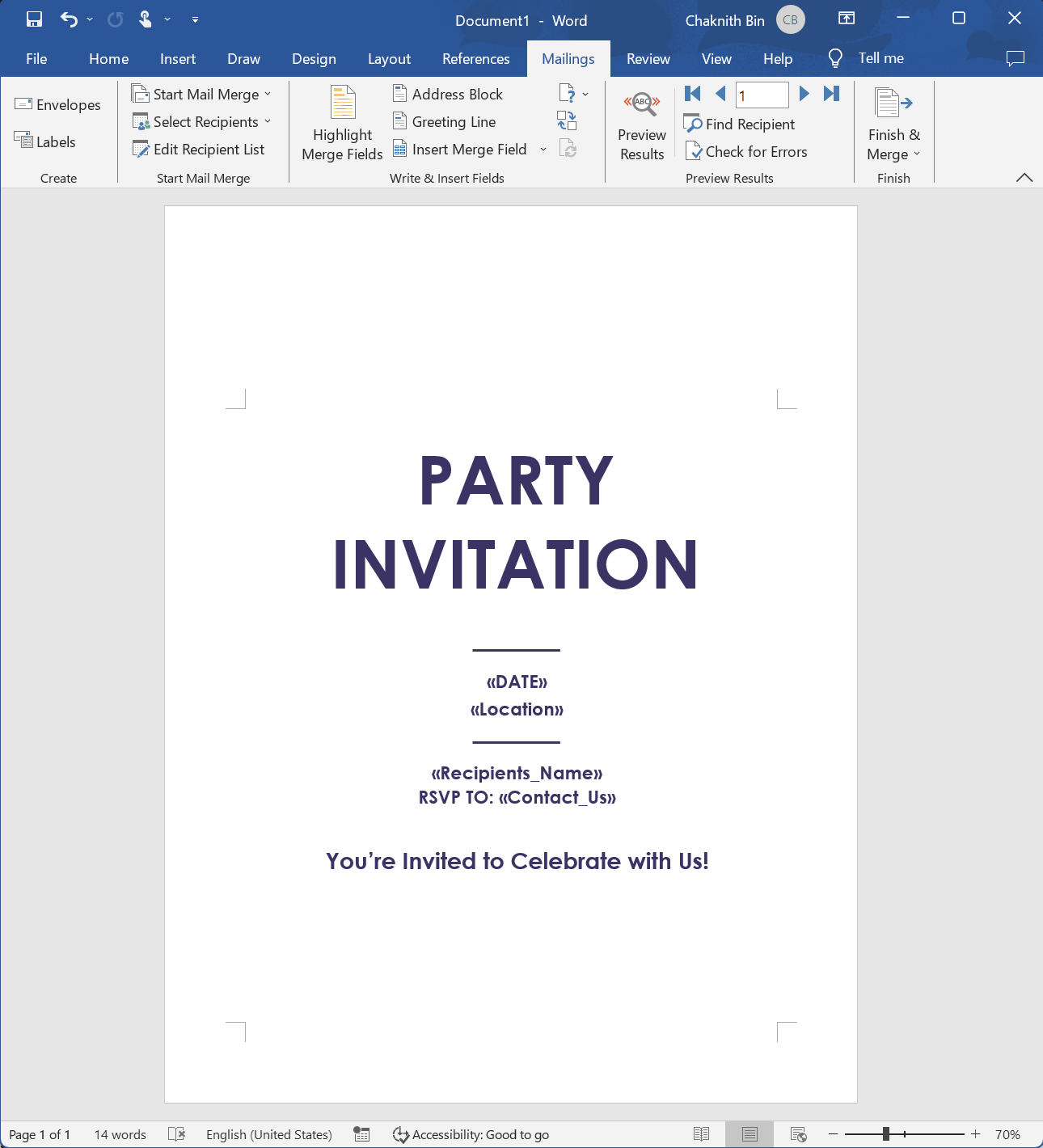
代码样本
:path=/static-assets/pdf/content-code-examples/how-to/docx-to-pdf-mail-merge.cs
using IronPdf;
using System.Collections.Generic;
using System.Linq;
var recipients = new List<RecipientsDataModel>()
{
new RecipientsDataModel()
{
Date ="Saturday, October 15th, 2023",
Location="Iron Software Cafe, Chiang Mai",
Recipients_Name="Olivia Smith",
Contact_Us = "support@ironsoftware.com"
},
new RecipientsDataModel()
{
Date ="Saturday, October 15th, 2023",
Location="Iron Software Cafe, Chiang Mai",
Recipients_Name="Ethan Davis",
Contact_Us = "support@ironsoftware.com"
},
};
DocxToPdfRenderer docxToPdfRenderer = new DocxToPdfRenderer();
// Apply render options
DocxPdfRenderOptions options = new DocxPdfRenderOptions();
// Configure the output PDF to be combined into a single PDF document
options.MailMergePrintAllInOnePdfDocument = true;
// Convert DOTX to PDF
var pdfs = docxToPdfRenderer.RenderDocxMailMergeAsPdf<RecipientsDataModel>(
recipients,
"Party-invitation.dotx",
options);
pdfs.First().SaveAs("mailMerge.pdf");
Imports IronPdf
Imports System.Collections.Generic
Imports System.Linq
Private recipients = New List(Of RecipientsDataModel)() From {
New RecipientsDataModel() With {
.Date ="Saturday, October 15th, 2023",
.Location="Iron Software Cafe, Chiang Mai",
.Recipients_Name="Olivia Smith",
.Contact_Us = "support@ironsoftware.com"
},
New RecipientsDataModel() With {
.Date ="Saturday, October 15th, 2023",
.Location="Iron Software Cafe, Chiang Mai",
.Recipients_Name="Ethan Davis",
.Contact_Us = "support@ironsoftware.com"
}
}
Private docxToPdfRenderer As New DocxToPdfRenderer()
' Apply render options
Private options As New DocxPdfRenderOptions()
' Configure the output PDF to be combined into a single PDF document
options.MailMergePrintAllInOnePdfDocument = True
' Convert DOTX to PDF
Dim pdfs = docxToPdfRenderer.RenderDocxMailMergeAsPdf(Of RecipientsDataModel)(recipients, "Party-invitation.dotx", options)
pdfs.First().SaveAs("mailMerge.pdf")
输出 PDF
创建PDF文档后,您可以灵活地进行额外的更改。 这些包括将其导出为PDF/A或PDF/UA以及添加一个数字证书. 您也可以操作单个页面通过合并或拆分 PDF并旋转它们,您还可以选择应用注释和书签.