如何將圖片轉換為 PDF
將圖片轉換為PDF是一個有用的過程,可以將多個圖像文件結合在一起(例如 JPG、PNG 或 TIFF)合併成一個PDF文件。 這通常用於創建數字化作品集、演示文稿或報告,使分享和存儲一系列圖像更加容易、有序且普遍可讀。
IronPDF 允許您將單張或多張圖片轉換為具有獨特性的 PDF 文件。圖片位置和行為. 這些行為包括適合頁面、頁面居中和裁剪頁面。 此外,您可以添加使用 IronPDF 的文字和 HTML 頁首和頁腳, 使用 IronPDF 套用浮水印設置自定義頁面尺寸,並包含背景和前景覆蓋。
開始使用 IronPDF
立即在您的專案中使用IronPDF,並享受免費試用。
如何將圖片轉換為 PDF
將圖像轉換為PDF示例
使用 ImageToPdfConverter 類別中的 ImageToPdf
靜態方法將圖像轉換為 PDF 文件。 此方法僅需圖像的檔案路徑,便能將其轉換成具有預設圖像放置和行為的PDF文件。 支持的圖片格式包括.bmp、.jpeg、.jpg、.gif、.png、.svg、.tif、.tiff、.webp、.apng、.avif、.cur、.dib、.ico、.jfif、.jif、.jpe、.pjp 和 .pjpeg。
範例圖片
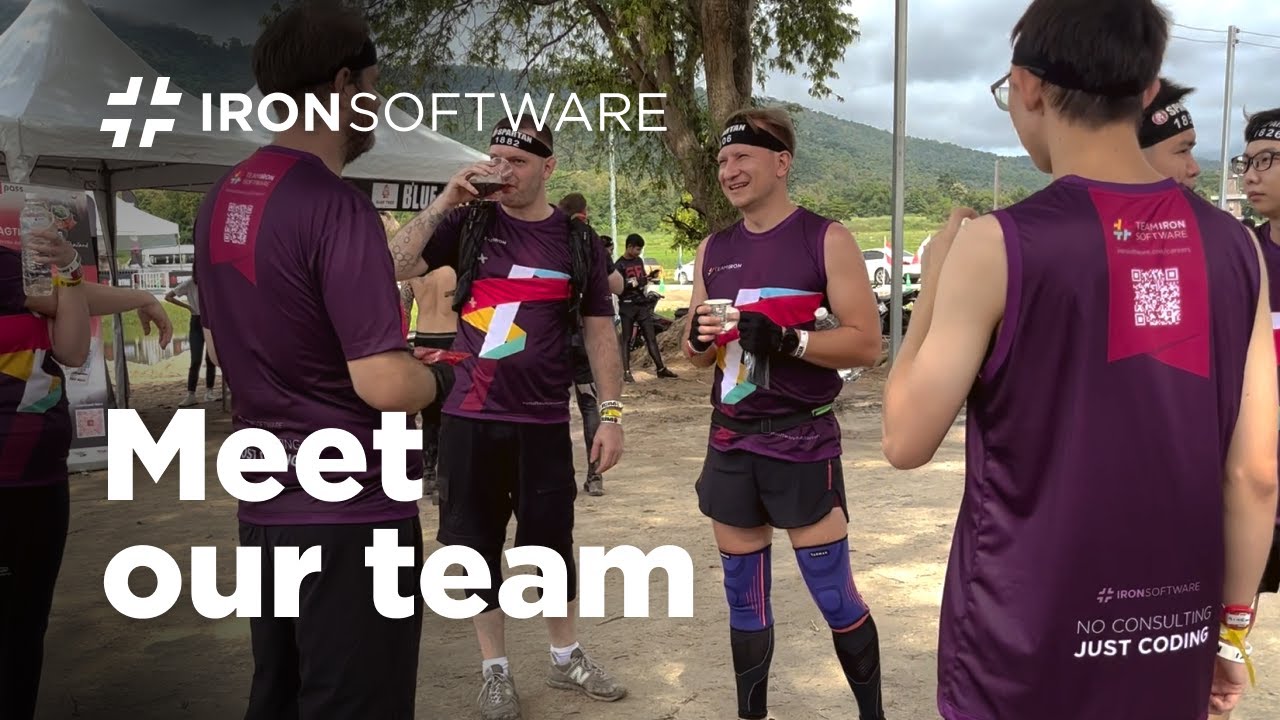
代碼
:path=/static-assets/pdf/content-code-examples/how-to/image-to-pdf-convert-one-image.cs
using IronPdf;
string imagePath = "meetOurTeam.jpg";
// Convert an image to a PDF
PdfDocument pdf = ImageToPdfConverter.ImageToPdf(imagePath);
// Export the PDF
pdf.SaveAs("imageToPdf.pdf");
Imports IronPdf
Private imagePath As String = "meetOurTeam.jpg"
' Convert an image to a PDF
Private pdf As PdfDocument = ImageToPdfConverter.ImageToPdf(imagePath)
' Export the PDF
pdf.SaveAs("imageToPdf.pdf")
輸出 PDF
將圖像轉換為PDF範例
要將多個圖像轉換成 PDF 文件,您應該提供一個包含文件路徑的 IEnumerable 對象,而不是像我們之前示例中的單個文件路徑。 這將再次生成一個具有預設圖像放置和行為的PDF文件。
:path=/static-assets/pdf/content-code-examples/how-to/image-to-pdf-convert-multiple-images.cs
using IronPdf;
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
// Retrieve all JPG and JPEG image paths in the 'images' folder.
IEnumerable<String> imagePaths = Directory.EnumerateFiles("images").Where(f => f.EndsWith(".jpg") || f.EndsWith(".jpeg"));
// Convert images to a PDF
PdfDocument pdf = ImageToPdfConverter.ImageToPdf(imagePaths);
// Export the PDF
pdf.SaveAs("imagesToPdf.pdf");
Imports IronPdf
Imports System
Imports System.Collections.Generic
Imports System.IO
Imports System.Linq
' Retrieve all JPG and JPEG image paths in the 'images' folder.
Private imagePaths As IEnumerable(Of String) = Directory.EnumerateFiles("images").Where(Function(f) f.EndsWith(".jpg") OrElse f.EndsWith(".jpeg"))
' Convert images to a PDF
Private pdf As PdfDocument = ImageToPdfConverter.ImageToPdf(imagePaths)
' Export the PDF
pdf.SaveAs("imagesToPdf.pdf")
輸出 PDF
圖片放置和行為
为了使用方便,我们提供了多种有用的图像放置和行为选项。 例如,您可以將圖像置於頁面中央,或者將其調整至頁面大小,同時保持其縱橫比。 所有可用的影像位置和行為如下:
- TopLeftCornerOfPage: 圖像放置在頁面的左上角。
- TopRightCornerOfPage: 圖像放置於頁面的右上角。
- CenteredOnPage: 圖像居中於頁面上。
- FitToPageAndMaintainAspectRatio: 圖片在保持其原始比例的同時適應頁面。
- BottomLeftCornerOfPage: 圖像放置於頁面的左下角。
- 頁面右下角:圖像放置在頁面的右下角。
- FitToPage: 圖片適合頁面。
- CropPage:頁面將根據圖像進行調整。
:path=/static-assets/pdf/content-code-examples/how-to/image-to-pdf-convert-one-image-image-behavior.cs
using IronPdf;
using IronPdf.Imaging;
string imagePath = "meetOurTeam.jpg";
// Convert an image to a PDF with image behavior of centered on page
PdfDocument pdf = ImageToPdfConverter.ImageToPdf(imagePath, ImageBehavior.CenteredOnPage);
// Export the PDF
pdf.SaveAs("imageToPdf.pdf");
Imports IronPdf
Imports IronPdf.Imaging
Private imagePath As String = "meetOurTeam.jpg"
' Convert an image to a PDF with image behavior of centered on page
Private pdf As PdfDocument = ImageToPdfConverter.ImageToPdf(imagePath, ImageBehavior.CenteredOnPage)
' Export the PDF
pdf.SaveAs("imageToPdf.pdf")
影像行為比較
![]() 頁面的左上角 | ![]() 頁面的右上角 |
![]() 頁面居中 | ![]() 適應頁面並保持縱橫比 |
![]() 頁面左下角 | ![]() 頁面右下角 |
![]() 縮放至頁面 | ![]() 裁剪頁面 |
套用渲染選項
在ImageToPdf
靜態方法的底層將各類型的圖像轉換為 PDF 文件的關鍵是將圖像作為 HTML 導入將 HTML 包含在 `
標籤中,然後將 HTML 轉換為 PDF。 這也是我們可以將 **ChromePdfRenderOptions** 物件作為第三個參數傳遞給
ImageToPdf` 方法以直接自定義渲染過程的原因。
:path=/static-assets/pdf/content-code-examples/how-to/image-to-pdf-convert-one-image-rendering-options.cs
using IronPdf;
string imagePath = "meetOurTeam.jpg";
ChromePdfRenderOptions options = new ChromePdfRenderOptions()
{
HtmlHeader = new HtmlHeaderFooter()
{
HtmlFragment = "<h1 style='color: #2a95d5;'>Content Header</h1>",
DrawDividerLine = true,
},
};
// Convert an image to a PDF with custom header
PdfDocument pdf = ImageToPdfConverter.ImageToPdf(imagePath, options: options);
// Export the PDF
pdf.SaveAs("imageToPdfWithHeader.pdf");
Imports IronPdf
Private imagePath As String = "meetOurTeam.jpg"
Private options As New ChromePdfRenderOptions() With {
.HtmlHeader = New HtmlHeaderFooter() With {
.HtmlFragment = "<h1 style='color: #2a95d5;'>Content Header</h1>",
.DrawDividerLine = True
}
}
' Convert an image to a PDF with custom header
Private pdf As PdfDocument = ImageToPdfConverter.ImageToPdf(imagePath, options:= options)
' Export the PDF
pdf.SaveAs("imageToPdfWithHeader.pdf")
輸出 PDF
如果您想將 PDF 文件轉換或光柵化為圖像,請參考我們的如何將 PDF 光柵化為圖像的指南.