如何在 C# 中將 Microsoft Word 轉換為 PDF
DOCX 文件是在 Microsoft Word 中創建的文件,Microsoft Word 是微軟的文字處理程序。 它使用 Office Open XML(OOXML)標準化,使其高效且與各種軟體兼容。 自 Word 2007 起,它成為 Word 文件的預設格式,取代了較舊的 DOC 格式。
IronPDF允許您將DOCX文件轉換為PDF,並提供郵件合併功能,用於為個別收件人生成個性化的文件批次。 將DOCX轉換為PDF可確保通用性,保留格式,並增加一層安全性。
開始使用 IronPDF
立即在您的專案中使用IronPDF,並享受免費試用。
如何在 C# 中將 DOCX 轉換為 PDF
- 下載將 DOCX 轉換為 PDF 的 C# 程式庫
- 準備您想要轉換的DOCX文件
- 實例化 DocxToPdfRenderer 從DOCX文件渲染PDF的類
- 使用
將Docx呈現為PDF
方法並提供 DOCX 文件路徑 - 利用郵件合併功能生成一批文件
將DOCX文件轉換為PDF示例
要將 Microsoft Word 檔案轉換成 PDF,請實例化 DocxToPdfRenderer 類別。 使用DocxToPdfRenderer對象的RenderDocxAsPdf
方法,提供DOCX文件的文件路徑。此方法返回一個PdfDocument對象,讓您可以進一步自訂PDF。 我使用了 Microsoft Word 中的「現代時間順序履歷」範本作為範例。 您可以下載該現代時序履歷DOCX範例文件.
Microsoft Word 預覽
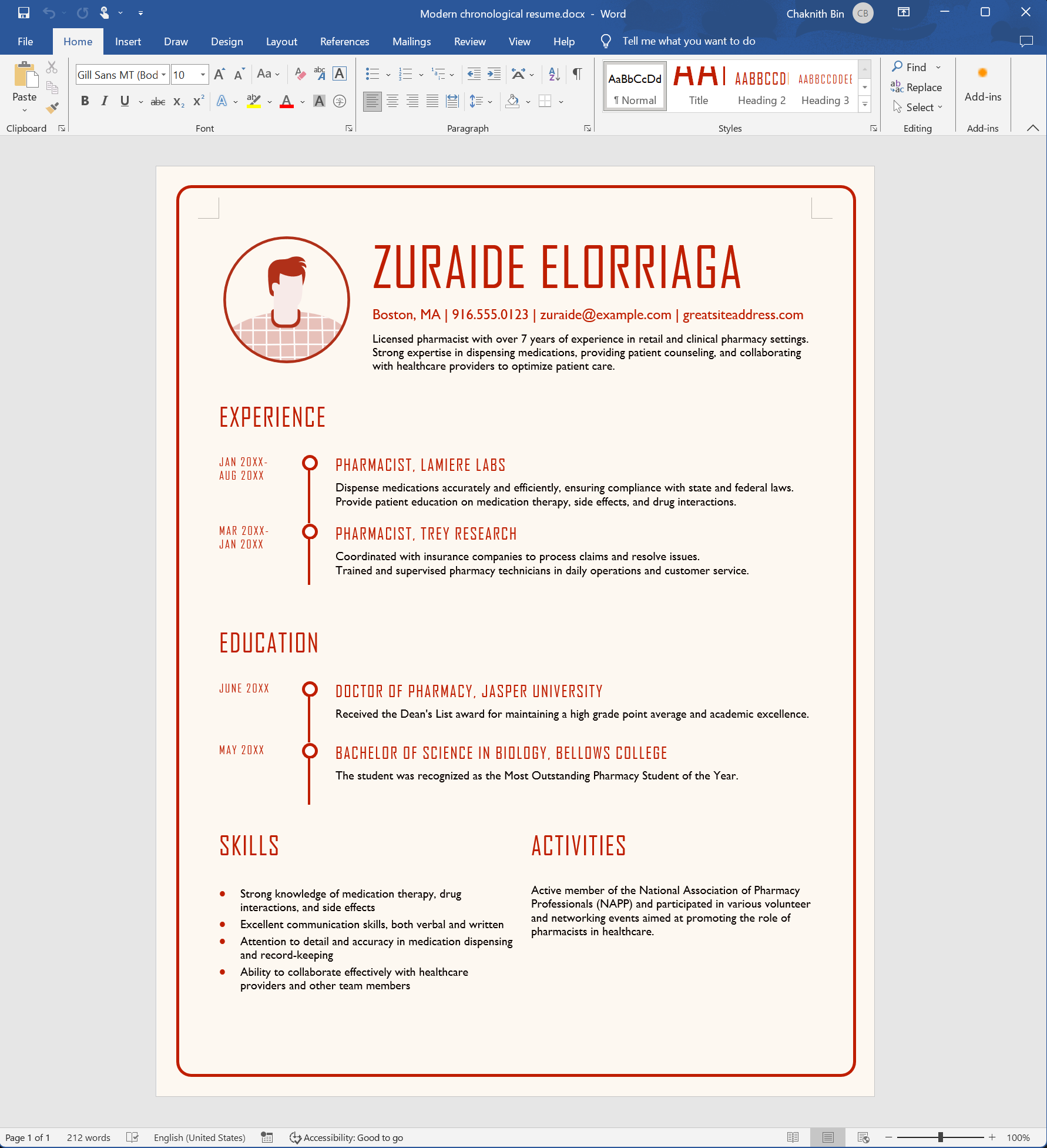
代碼範例
此外,RenderDocxAsPdf
方法也接受以字節和流的形式的 DOCX 數據。
:path=/static-assets/pdf/content-code-examples/how-to/docx-to-pdf-from-file.cs
using IronPdf;
// Instantiate Renderer
DocxToPdfRenderer renderer = new DocxToPdfRenderer();
// Render from DOCX file
PdfDocument pdf = renderer.RenderDocxAsPdf("Modern-chronological-resume.docx");
// Save the PDF
pdf.SaveAs("pdfFromDocx.pdf");
Imports IronPdf
' Instantiate Renderer
Private renderer As New DocxToPdfRenderer()
' Render from DOCX file
Private pdf As PdfDocument = renderer.RenderDocxAsPdf("Modern-chronological-resume.docx")
' Save the PDF
pdf.SaveAs("pdfFromDocx.pdf")
輸出 PDF
郵件合併示例
郵件合併位於 Microsoft Word 中的「郵件」標籤,允許您為每個收件人或數據條目創建一批帶有個人化信息的文件。 它通常用於生成個性化的信件、信封、標籤或電子郵件訊息,例如邀請函、通訊稿或表格信件,其中大部分內容相同,但某些細節會因每個收件人而有所不同。
模型
首先,讓我們建立一個模型來儲存將被郵件合併到相應占位符中的資訊。
:path=/static-assets/pdf/content-code-examples/how-to/docx-to-pdf-mail-merge-model.cs
internal class RecipientsDataModel
{
public string Date { get; set; }
public string Location{ get; set; }
public string Recipients_Name { get; set; }
public string Contact_Us { get; set; }
}
Friend Class RecipientsDataModel
Public Property [Date]() As String
Public Property Location() As String
Public Property Recipients_Name() As String
Public Property Contact_Us() As String
End Class
我已經修改了微著提供的模板以符合我們的需求。 請下載Party Invitation DOTX 範例檔案. 對於我們的使用案例,讓我們將 MailMergePrintAllInOnePdfDocument 屬性設定為 true,這會將 PDF 合併為一個 PdfDocument 物件。 我們將要使用的合併欄位有日期、地點、收件人姓名和聯絡我們。
Microsoft Word 預覽
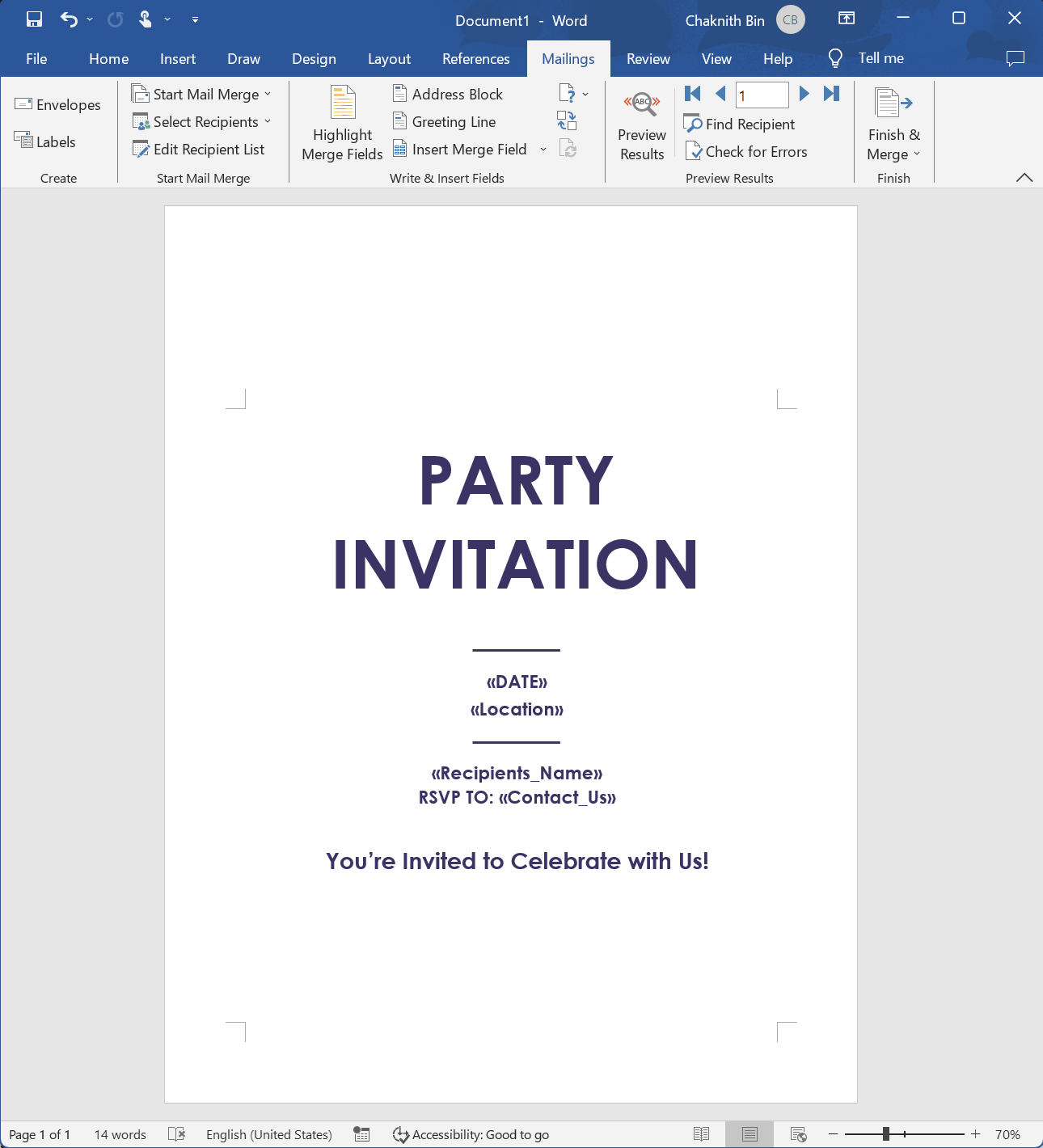
代碼範例
:path=/static-assets/pdf/content-code-examples/how-to/docx-to-pdf-mail-merge.cs
using IronPdf;
using System.Collections.Generic;
using System.Linq;
var recipients = new List<RecipientsDataModel>()
{
new RecipientsDataModel()
{
Date ="Saturday, October 15th, 2023",
Location="Iron Software Cafe, Chiang Mai",
Recipients_Name="Olivia Smith",
Contact_Us = "support@ironsoftware.com"
},
new RecipientsDataModel()
{
Date ="Saturday, October 15th, 2023",
Location="Iron Software Cafe, Chiang Mai",
Recipients_Name="Ethan Davis",
Contact_Us = "support@ironsoftware.com"
},
};
DocxToPdfRenderer docxToPdfRenderer = new DocxToPdfRenderer();
// Apply render options
DocxPdfRenderOptions options = new DocxPdfRenderOptions();
// Configure the output PDF to be combined into a single PDF document
options.MailMergePrintAllInOnePdfDocument = true;
// Convert DOTX to PDF
var pdfs = docxToPdfRenderer.RenderDocxMailMergeAsPdf<RecipientsDataModel>(
recipients,
"Party-invitation.dotx",
options);
pdfs.First().SaveAs("mailMerge.pdf");
Imports IronPdf
Imports System.Collections.Generic
Imports System.Linq
Private recipients = New List(Of RecipientsDataModel)() From {
New RecipientsDataModel() With {
.Date ="Saturday, October 15th, 2023",
.Location="Iron Software Cafe, Chiang Mai",
.Recipients_Name="Olivia Smith",
.Contact_Us = "support@ironsoftware.com"
},
New RecipientsDataModel() With {
.Date ="Saturday, October 15th, 2023",
.Location="Iron Software Cafe, Chiang Mai",
.Recipients_Name="Ethan Davis",
.Contact_Us = "support@ironsoftware.com"
}
}
Private docxToPdfRenderer As New DocxToPdfRenderer()
' Apply render options
Private options As New DocxPdfRenderOptions()
' Configure the output PDF to be combined into a single PDF document
options.MailMergePrintAllInOnePdfDocument = True
' Convert DOTX to PDF
Dim pdfs = docxToPdfRenderer.RenderDocxMailMergeAsPdf(Of RecipientsDataModel)(recipients, "Party-invitation.dotx", options)
pdfs.First().SaveAs("mailMerge.pdf")
輸出 PDF
一旦PDF文件創建後,您可以進行額外的修改。 這些包括將其匯出為PDF/A或PDF/UA,並添加一个數位憑證. 您也可以操作個別頁面。合併或拆分PDF檔案, 並旋轉它們,您可以選擇應用註解和書籤.