How to Render HTML File to PDF
One of the easiest ways to use IronPDF is to tell it to render an HTML file. IronPDF can render any HTML file that the machine has access to.
Get started with IronPDF
Start using IronPDF in your project today with a free trial.
How to Render HTML File to PDF
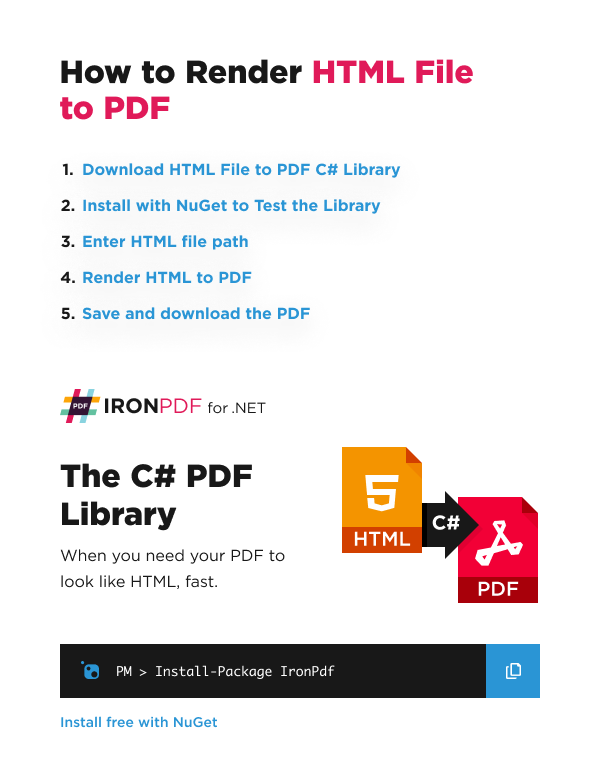
- Download IronPDF Library for HTML to PDF Conversion
- Instantiate the ChromePdfRenderer class
- Configure the RenderingOptions to fine-tune the output PDF
- Pass the HTML file path to the renderer
- Save and download the PDF
Convert HTML to PDF Example
Here we have an example of IronPDF rendering an HTML file into a PDF by using the RenderHtmlFileAsPdf()
method. The parameter is a filepath to a local HTML file.
This method has the advantage of allowing the developer the opportunity to test the HTML content in a browser during development. They can, in particular, test the fidelity in rendering. We recommend Chrome, as it is the web browser on which IronPDF's rendering engine is based.
If it looks right in Chrome, then it will be pixel-perfect in IronPDF as well.
Input File
This is the example.html
HTML file that the code renders:
:path=/static-assets/pdf/how-to/html-file-to-pdf/example.html
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
</head>
<body>
<h1>My First Heading</h1>
<p>My first paragraph.</p>
</body>
</html>
The HTML file rendered on the web is displayed below.
Code Example
:path=/static-assets/pdf/content-code-examples/how-to/html-file-to-pdf.cs
using IronPdf;
using IronPdf.Engines.Chrome;
using IronPdf.Rendering;
var renderer = new ChromePdfRenderer
{
RenderingOptions = new ChromePdfRenderOptions
{
CssMediaType = PdfCssMediaType.Print,
MarginBottom = 0,
MarginLeft = 0,
MarginRight = 0,
MarginTop = 0,
Timeout = 120,
},
};
renderer.RenderingOptions.WaitFor.RenderDelay(50);
// Create a PDF from an existing HTML file using C#
var pdf = renderer.RenderHtmlFileAsPdf("example.html");
// Export to a file or Stream
pdf.SaveAs("output.pdf");
Imports IronPdf
Imports IronPdf.Engines.Chrome
Imports IronPdf.Rendering
Private renderer = New ChromePdfRenderer With {
.RenderingOptions = New ChromePdfRenderOptions With {
.CssMediaType = PdfCssMediaType.Print,
.MarginBottom = 0,
.MarginLeft = 0,
.MarginRight = 0,
.MarginTop = 0,
.Timeout = 120
}
}
renderer.RenderingOptions.WaitFor.RenderDelay(50)
' Create a PDF from an existing HTML file using C#
Dim pdf = renderer.RenderHtmlFileAsPdf("example.html")
' Export to a file or Stream
pdf.SaveAs("output.pdf")
Result
This is the PDF file that the code produced:
Default Chrome Print Options
In the case that a default Chrome Print Options is desired, access the DefaultChrome property of the ChromePdfRenderOptions class and assign it to the RenderingOptions. With this setting, the PDF output from IronPdf will be identical to the Chrome Print Preview.
:path=/static-assets/pdf/content-code-examples/how-to/html-file-to-pdf-default-chrome.cs
using IronPdf;
ChromePdfRenderer renderer = new ChromePdfRenderer();
// Configure the rendering options to default Chrome options
renderer.RenderingOptions = ChromePdfRenderOptions.DefaultChrome;
Imports IronPdf
Private renderer As New ChromePdfRenderer()
' Configure the rendering options to default Chrome options
renderer.RenderingOptions = ChromePdfRenderOptions.DefaultChrome